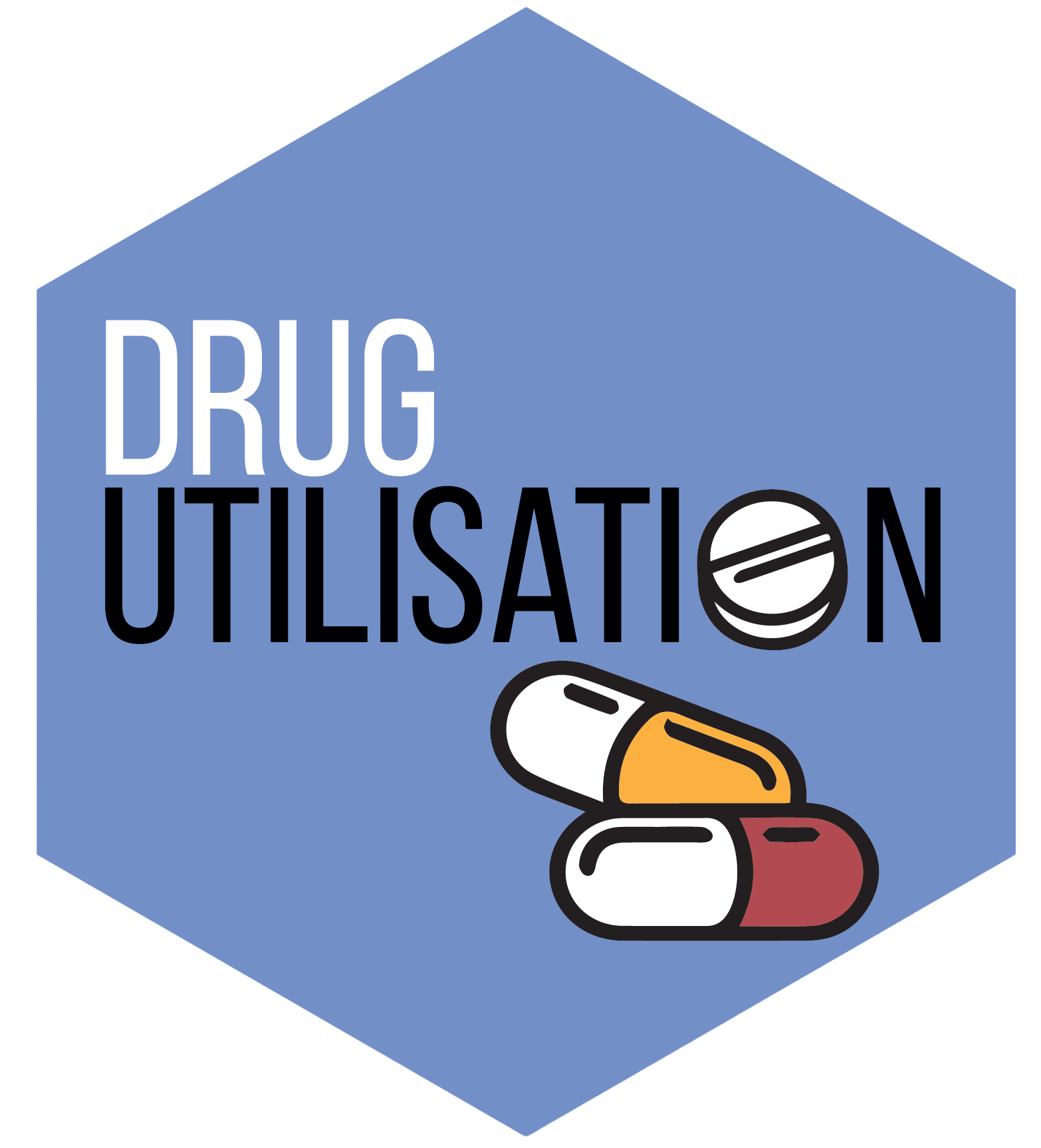
Use DrugUtilisation to create a cohort
Marti Catala, Mike Du, Yuchen Guo, Kim Lopez-Guell, Edward Burn, Xintong Li
2024-07-16
a01_introCreateCohort.Rmd
Introduction
In this vignette we will introduce different ways to create a drug utilisation cohort using DrugUtilisation.
Create a cdm object
To create a cdm_reference object you will need to use the package CDMConnector. For this vignette we will use mock data contained in the DrugUtilisation package.
library(DrugUtilisation)
library(dplyr)
library(CDMConnector)
cdm <- mockDrugUtilisation(numberIndividual = 200)
Get the concept code
The first thing that we need is a concept list. The concept list can be obtained through different ways:
- Read from .json file
- Use concept code directly
- Use CodelistGenerator
Concept list from a .json file
To create a cohort with a concept list from a .json file, use
codesFromConceptSet()
from CodelistGenerator package. Let’s
see an example:
conceptSet_json <- codesFromConceptSet(here::here("inst/Concept"), cdm)
conceptSet_json
#>
#> ── 1 codelist ──────────────────────────────────────────────────────────────────
#>
#> - asthma (1 codes)
Concept list listed directly
The concept list can also be created manually:
#get concept using code directly
conceptSet_code <- list(asthma = 317009)
conceptSet_code
#> $asthma
#> [1] 317009
Concept list of an ingredient
In case there is a certain ingredient of interest, the code can also
be obtained by getDrugIngredientCodes()
from the package
CodelistGenerator.
#get concept by ingredient
conceptSet_ingredient <- getDrugIngredientCodes(cdm, name = "simvastatin")
conceptSet_ingredient
#>
#> - 36567_simvastatin (3 codes)
Concept list from an ATC code
We can also obtain the ATC code by using getATCCodes()
from CodelistGenerator package.
#get concept from ATC codes
conceptSet_ATC <- getATCCodes(cdm,
level = "ATC 1st",
name = "ALIMENTARY TRACT AND METABOLISM")
conceptSet_ATC
#>
#> - A_alimentary_tract_and_metabolism (1 codes)
Create a cohort
Once we have the conceptSet
, we can proceed to generate
a cohort. There are two functions in this package to do that:
CDMConnector::generateConceptCohortSet()
: to generate a cohort for a certain list of concepts (they do not have to be a drug). This function is exported from CDMConnector package.generateDrugUtilisationCohortSet()
: to generate a cohort of the drug use.
Use CDMConnector::generateConceptCohortSet() to create a cohort
Let’s try to use generateConceptCohortSet()
to get the
asthma cohort using the conceptSet_code
created before. We
could also use conceptSet_json_1
or
conceptSet_json_2
to obtain the same result.
cdm <- CDMConnector::generateConceptCohortSet(cdm,
conceptSet = conceptSet_code,
name = "asthma_1",
overwrite = TRUE
)
cdm$asthma_1
#> # Source: table<asthma_1> [?? x 4]
#> # Database: DuckDB v1.0.0 [unknown@Linux 6.5.0-1023-azure:R 4.4.1/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date
#> <int> <int> <date> <date>
#> 1 1 10 2004-03-15 2015-08-30
#> 2 1 156 2017-12-01 2021-08-08
#> 3 1 158 2020-09-03 2020-10-10
#> 4 1 143 2017-12-15 2018-05-22
#> 5 1 184 2012-04-11 2013-01-04
#> 6 1 122 2018-10-16 2020-08-01
#> 7 1 74 2019-04-13 2019-10-25
#> 8 1 21 1982-04-22 2003-07-18
#> 9 1 25 2001-06-29 2016-10-18
#> 10 1 99 1989-03-11 1990-03-30
#> # ℹ more rows
The count of the cohort can be assessed using
cohortCount()
from CDMConnector.
cohortCount(cdm$asthma_1)
#> # A tibble: 1 × 3
#> cohort_definition_id number_records number_subjects
#> <int> <int> <int>
#> 1 1 100 100
Cohort attrition can be assessed using attrition()
from
CDMConnector.
attrition(cdm$asthma_1)
#> # A tibble: 1 × 7
#> cohort_definition_id number_records number_subjects reason_id reason
#> <int> <int> <int> <int> <chr>
#> 1 1 100 100 1 Initial qualify…
#> # ℹ 2 more variables: excluded_records <int>, excluded_subjects <int>
end parameter
You can use the end
parameter to set how the cohort end
date will be defined. By default,
end = observation_period_end_date
, but it can also be
defined as event_end_date
or by defining a numeric scalar.
See an example below:
cdm <- CDMConnector::generateConceptCohortSet(cdm,
conceptSet = conceptSet_code,
name = "asthma_2",
end = "event_end_date",
overwrite = TRUE
)
cdm$asthma_2
#> # Source: table<asthma_2> [?? x 4]
#> # Database: DuckDB v1.0.0 [unknown@Linux 6.5.0-1023-azure:R 4.4.1/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date
#> <int> <int> <date> <date>
#> 1 1 21 1982-04-22 1991-04-15
#> 2 1 25 2001-06-29 2015-02-20
#> 3 1 99 1989-03-11 1989-11-22
#> 4 1 148 2011-05-24 2017-02-01
#> 5 1 122 2018-10-16 2019-04-13
#> 6 1 6 2021-11-24 2022-01-17
#> 7 1 136 2004-07-05 2005-08-19
#> 8 1 85 1998-12-22 2005-06-03
#> 9 1 96 2019-08-18 2020-06-03
#> 10 1 117 1991-11-16 1993-05-08
#> # ℹ more rows
requiredObservation parameter
The requiredObservation
parameter is a numeric vector of
length 2, that defines the number of days of required observation time
prior to index and post index for an event to be included in the cohort.
The default value is c(0,0)
. Let’s check how the difference
between asthma_3 and asthma_2 when
changing this parameter.
cdm <- CDMConnector::generateConceptCohortSet(cdm,
conceptSet = conceptSet_code,
name = "asthma_3",
end = "observation_period_end_date",
requiredObservation = c(10, 10),
overwrite = TRUE
)
cdm$asthma_3
#> # Source: table<asthma_3> [?? x 4]
#> # Database: DuckDB v1.0.0 [unknown@Linux 6.5.0-1023-azure:R 4.4.1/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date
#> <int> <int> <date> <date>
#> 1 1 105 2018-11-29 2021-02-12
#> 2 1 178 2006-06-19 2010-11-06
#> 3 1 138 2006-06-10 2007-06-10
#> 4 1 96 2019-08-18 2020-07-02
#> 5 1 168 2020-05-04 2020-05-15
#> 6 1 191 2018-02-06 2018-05-19
#> 7 1 199 1996-10-11 2014-04-23
#> 8 1 57 2015-06-05 2017-03-14
#> 9 1 98 2017-05-03 2018-11-21
#> 10 1 17 2017-07-25 2022-06-10
#> # ℹ more rows
cohortCount(cdm$asthma_3)
#> # A tibble: 1 × 3
#> cohort_definition_id number_records number_subjects
#> <int> <int> <int>
#> 1 1 94 94
attrition(cdm$asthma_3)
#> # A tibble: 1 × 7
#> cohort_definition_id number_records number_subjects reason_id reason
#> <int> <int> <int> <int> <chr>
#> 1 1 94 94 1 Initial qualify…
#> # ℹ 2 more variables: excluded_records <int>, excluded_subjects <int>
Use generateDrugUtilisationCohortSet() to generate a cohort
Now let’s try the function
generateDrugUtilisationCohortSet()
to get the drug cohort
for the ingredient simvastatin. See an example below:
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_1",
conceptSet = conceptSet_ingredient
)
cdm$simvastin_1
#> # Source: SQL [?? x 4]
#> # Database: DuckDB v1.0.0 [unknown@Linux 6.5.0-1023-azure:R 4.4.1/:memory:]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date
#> <int> <int> <date> <date>
#> 1 1 99 1989-05-19 1989-08-06
#> 2 1 148 2008-05-26 2011-10-10
#> 3 1 151 1999-07-08 2002-06-20
#> 4 1 176 2008-11-03 2008-11-03
#> 5 1 15 2009-04-20 2010-01-01
#> 6 1 166 2019-02-05 2020-05-06
#> 7 1 180 2020-12-15 2021-07-15
#> 8 1 38 2012-12-17 2013-01-12
#> 9 1 152 2016-10-27 2021-02-08
#> 10 1 111 2015-06-18 2015-08-17
#> # ℹ more rows
cohortCount(cdm$simvastin_1)
#> # A tibble: 1 × 3
#> cohort_definition_id number_records number_subjects
#> <int> <int> <int>
#> 1 1 109 99
attrition(cdm$simvastin_1)
#> # A tibble: 2 × 7
#> cohort_definition_id number_records number_subjects reason_id reason
#> <int> <int> <int> <int> <chr>
#> 1 1 109 99 1 Initial qualify…
#> 2 1 109 99 2 join exposures …
#> # ℹ 2 more variables: excluded_records <int>, excluded_subjects <int>
imputeDuration and durationRange parameters
The parameterdurationRange
specifies the range within
which the duration must fall, where duration will be calculated as:
duration = cohort_end_date - cohort_start_date + 1
The default value is c(1, Inf)
. See that this parameter
must be a numeric vector of length two, with no NAs and with the first
value equal or bigger than the second one. Duration values outside of
durationRange
will be imputed using
imputeDuration
. imputeDuration
can be set as:
none
(default), median
, mean
,
mode
or an integer (count).
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_2",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf) # default as c(1, Inf)
)
attrition(cdm$simvastin_2)
#> # A tibble: 2 × 7
#> cohort_definition_id number_records number_subjects reason_id reason
#> <int> <int> <int> <int> <chr>
#> 1 1 109 99 1 Initial qualify…
#> 2 1 109 99 2 join exposures …
#> # ℹ 2 more variables: excluded_records <int>, excluded_subjects <int>
gapEra paratemer
The gapEra
parameter defines the number of days between
two continuous drug exposures to be considered as a same era. Now let’s
change it from 0 to a larger number to see what happens.
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_3",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 30 # default as 0
)
attrition(cdm$simvastin_3) |> select(number_records, reason, excluded_records, excluded_subjects)
#> # A tibble: 2 × 4
#> number_records reason excluded_records excluded_subjects
#> <int> <chr> <int> <int>
#> 1 109 Initial qualifying events 0 0
#> 2 107 join exposures separated by… 2 0
From the simvastin_3 cohort attrition, we can see that when joining eras, it resulted in less records, compared to the simvastin_2 cohort, as exposures with less than 30 days gaps are joined.
priorUseWashout parameter
The priorUseWashout
parameter specifies the number of
prior days without exposure (often termed as ‘washout’) that are
required. By default, it is set to NULL, meaning no washout period is
necessary. See that when increasing this value, the number of records
decrease.
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_4",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 30,
priorUseWashout = 30
)
attrition(cdm$simvastin_4) |> select(number_records, reason, excluded_records, excluded_subjects)
#> # A tibble: 2 × 4
#> number_records reason excluded_records excluded_subjects
#> <int> <chr> <int> <int>
#> 1 109 Initial qualifying events 0 0
#> 2 107 join exposures separated by… 2 0
priorObservation parameter
The parameter priorObservation
defines the minimum
number of days of prior observation necessary for drug eras to be taken
into account. If set to NULL, the drug eras are not required to fall
within the observation_period.
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_5",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 30,
priorUseWashout = 30,
priorObservation = 30
)
attrition(cdm$simvastin_5) |> select(number_records, reason, excluded_records, excluded_subjects)
#> # A tibble: 2 × 4
#> number_records reason excluded_records excluded_subjects
#> <int> <chr> <int> <int>
#> 1 109 Initial qualifying events 0 0
#> 2 107 join exposures separated by… 2 0
cohortDateRange parameter
The cohortDateRange
parameter defines the range for the
cohort_start_date and cohort_end_date.
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_6",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 30,
priorUseWashout = 30,
priorObservation = 30,
cohortDateRange = as.Date(c("2010-01-01", "2011-01-01"))
)
attrition(cdm$simvastin_6) |> select(number_records, reason, excluded_records, excluded_subjects)
#> # A tibble: 2 × 4
#> number_records reason excluded_records excluded_subjects
#> <int> <chr> <int> <int>
#> 1 109 Initial qualifying events 0 0
#> 2 107 join exposures separated by… 2 0
limit parameter
The input limit
allows all
(default) and
first
options. If we set it to first
, we will
only obtain the first record that fulfills all the criteria. Observe how
it impacts the attrition of the simvastin_7 in
comparison to the simvastin_6 cohort. The number of
records has decreased because of the First
limit.
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_7",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 30,
priorUseWashout = 30,
priorObservation = 30,
cohortDateRange = as.Date(c("2010-01-01", "2011-01-01")),
limit = "First"
)
attrition(cdm$simvastin_7) |> select(number_records, reason, excluded_records, excluded_subjects)
#> # A tibble: 2 × 4
#> number_records reason excluded_records excluded_subjects
#> <int> <chr> <int> <int>
#> 1 109 Initial qualifying events 0 0
#> 2 107 join exposures separated by… 2 0
If we just wanted to get the first-ever era, we can also use this parameter. To achieve that, try the following setting:
cdm <- generateDrugUtilisationCohortSet(cdm,
name = "simvastin_8",
conceptSet = conceptSet_ingredient,
imputeDuration = "none",
durationRange = c(0, Inf),
gapEra = 0,
priorUseWashout = Inf,
priorObservation = 0,
cohortDateRange = as.Date(c(NA, NA)),
limit = "First"
)
Constructing concept sets and generating various cohorts are the initial steps in conducting a drug utilisation study. For further guidance on using getting more information like characteristics from these cohorts, please refer to the other vignettes.