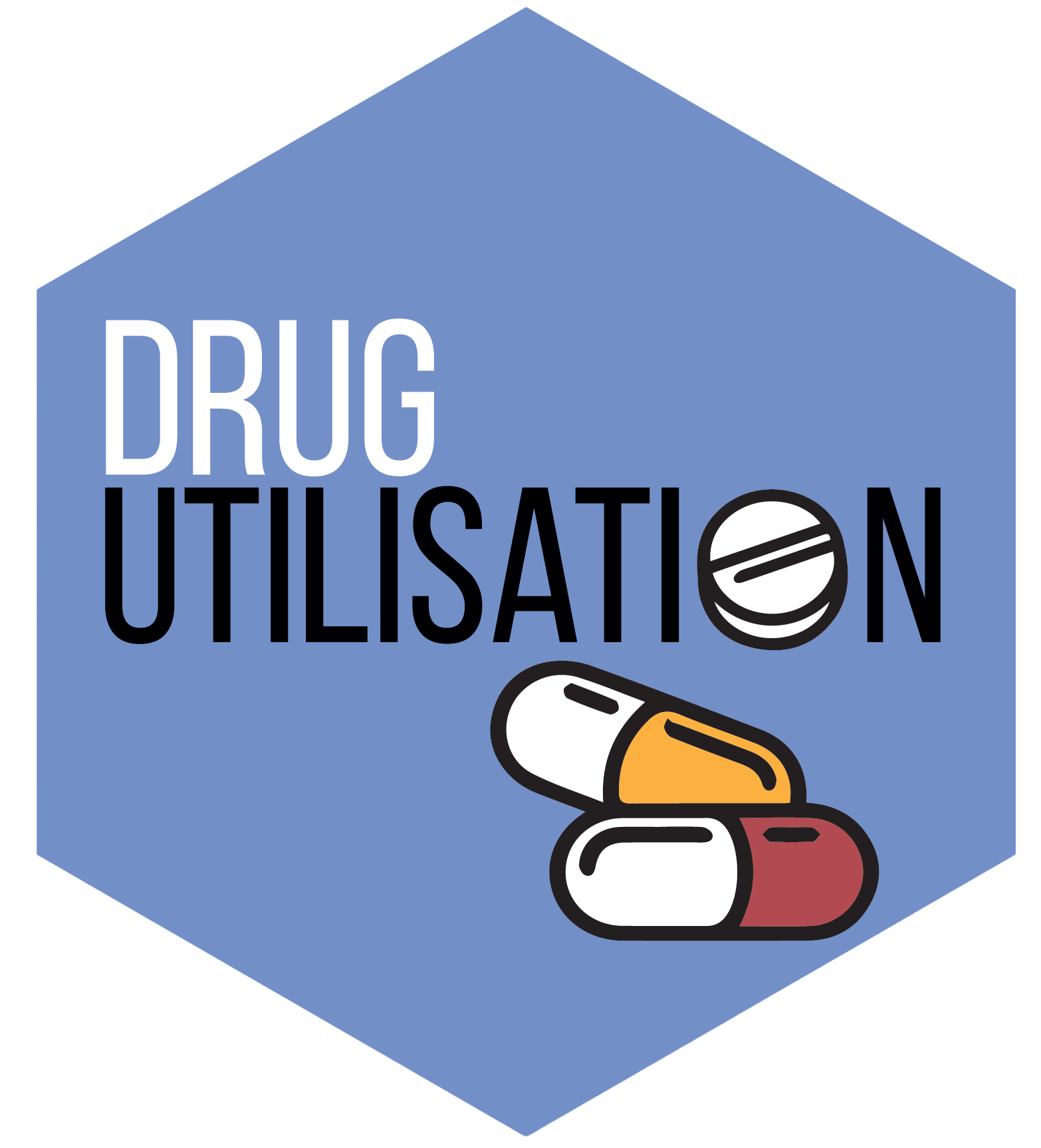
Add indications to Drug Utilisation Cohort
Marti Catala, Mike Du, Yuchen Guo, Kim Lopez-Guell, Edward Burn, Xintong Li
2024-07-17
a03_addIndications-example.Rmd
Introduction
In this vignette, we demonstrate how to use the
addIndications()
function to establish a binary indicator
between the drug utilisation cohort and another concept-based
cohort.
The DrugUtilisation package is designed to work with data in the OMOP CDM format, so our first step is to create a reference to the data using the DBI and CDMConnector packages. The connection to a Postgres database would look like:
library(DrugUtilisation)
library(DBI)
library(duckdb)
library(CDMConnector)
library(dplyr)
library(PatientProfiles)
con <- DBI::dbConnect(duckdb::duckdb(), eunomia_dir())
cdm <- CDMConnector::cdm_from_con(
con = con,
cdm_schema = "main",
write_schema = "main"
)
Create a drug utilisation cohort
We will use Acetaminophen as our example drug to construct our drug utilisation cohort. To begin, we’ll employ the CodelistGenerator package to generate a concept list associated with Acetaminophen.
conceptList <- CodelistGenerator::getDrugIngredientCodes(cdm, "acetaminophen")
conceptList
#>
#> - 161_acetaminophen (7 codes)
Next, we can create a drug utilisation cohort by using the
conceptList
with the
generateDrugUtilisationCohortSet()
function. For a better
understanding of the arguments and functionalities of
generateDrugUtilisationCohortSet()
, please refer to the
Use DrugUtilisation to create a cohort vignette.
cdm <- generateDrugUtilisationCohortSet(
cdm = cdm,
name = "acetaminophen_users",
conceptSet = conceptList,
limit = "All",
gapEra = 30,
priorUseWashout = 0
)
Create a indication cohort
Next we going to create our indications cohort to indicate patients
with sinusitis and bronchitis. This can be done by using
CDMConnector::generateConceptCohortSet()
.
indications <-
list(
sinusitis = c(257012, 4294548, 40481087),
bronchitis = c(260139, 258780)
)
cdm <-
CDMConnector::generateConceptCohortSet(cdm, name = "indications_cohort", indications)
cohortCount(cdm[["indications_cohort"]]) |>
left_join(
settings(cdm[["indications_cohort"]]) |>
select(cohort_definition_id, cohort_name),
by = "cohort_definition_id"
)
#> # A tibble: 2 × 4
#> cohort_definition_id number_records number_subjects cohort_name
#> <int> <int> <int> <chr>
#> 1 1 2688 2688 sinusitis
#> 2 2 2546 2546 bronchitis
Add indications with addIndication() function
Then to add indication to the drug utilisation cohort we can simple
use the addIndication()
function. To do that, we only need
the drug utilisation cohort and the indicationCohortName
.
However, other arguments can be specified using the additional
parameters. An example is provided below.
cdm[["acetaminophen_users"]] |>
addIndication(
indicationCohortName = "indications_cohort",
indicationWindow = list(c(0,0),c(-30,0),c(-365,0)),
unknownIndicationTable = c("condition_occurrence")
)
#> # Source: table<og_006_1721204179> [?? x 7]
#> # Database: DuckDB v1.0.0 [unknown@Linux 6.5.0-1023-azure:R 4.4.1//tmp/RtmprJVhK8/file1d5668e77d98.duckdb]
#> cohort_definition_id subject_id cohort_start_date cohort_end_date
#> <int> <int> <date> <date>
#> 1 1 3408 1997-01-13 1997-01-20
#> 2 1 114 1999-11-18 1999-12-02
#> 3 1 2945 1964-11-05 1964-11-12
#> 4 1 4370 1968-08-30 1968-09-13
#> 5 1 712 2011-07-27 2011-08-03
#> 6 1 5274 1953-09-29 1953-10-06
#> 7 1 565 1960-12-07 1960-12-14
#> 8 1 2380 1950-06-28 1950-07-12
#> 9 1 99 1980-11-30 1980-12-14
#> 10 1 219 1968-08-28 1968-09-11
#> # ℹ more rows
#> # ℹ 3 more variables: indication_0_to_0 <chr>, indication_m30_to_0 <chr>,
#> # indication_m365_to_0 <chr>
Use indicationGap
to specify the indication gaps, which
are defined as the gap between the event and the indication.
Additionally, you can use unknownIndicationTable
to specify
the tables to look for unknown indication.
Summarise indications with summariseIndication()
To create a summary table of the indications cohort, you can use the
summariseIndication()
function.
# cdm[["acetaminophen_users"]] |>
# addIndication(
# cdm = cdm,
# indicationCohortName = "indications_cohort",
# indicationGap = c(0, 30, 365),
# unknownIndicationTable = c("condition_occurrence")
# ) |>
# summariseIndication(cdm) |>
# select("variable_name", "estimate_name", "estimate_value")
You can also summarise the indications by using the
strata
argument in the summariseIndication()
function. In the example below, it is summarized by
ageGroup
and sex
.
# cdm[["acetaminophen_users"]] |>
# addDemographics(ageGroup = list(c(0, 19), c(20, 150))) |>
# addIndication(
# cdm = cdm,
# indicationCohortName = "indications_cohort",
# indicationGap = c(0),
# unknownIndicationTable = c("condition_occurrence")
# ) |>
# summariseIndication(
# cdm,
# strata = list("age" = "age_group", "sex" = "sex")) |>
# select("variable_name", "estimate_name", "estimate_value","strata_name")