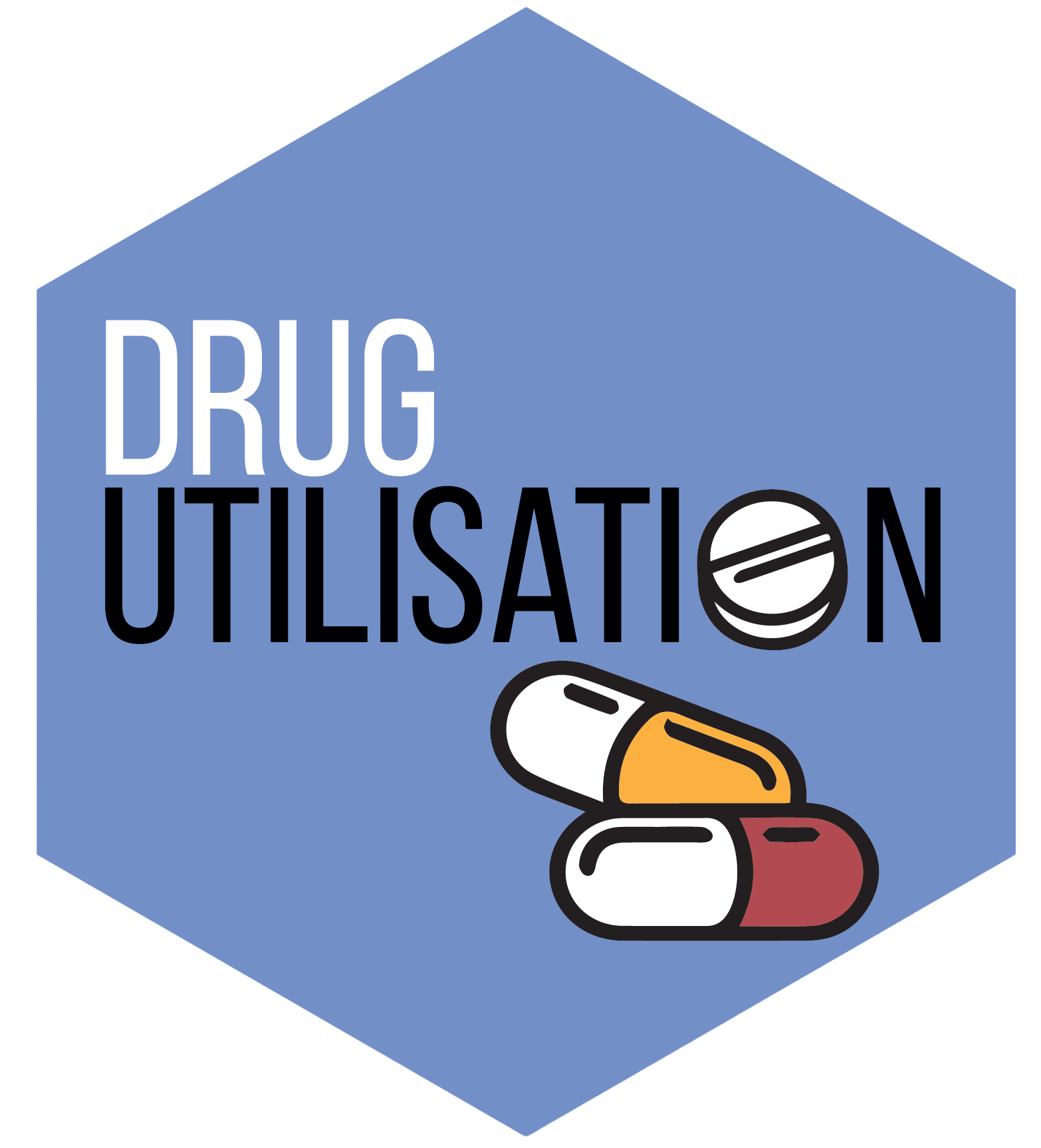
Add treatment summary to a Drug Utilisation cohort
Marti Catala, Mike Du, Yuchen Guo, Kim Lopez-Guell, Edward Burn, Xintong Li
2024-07-17
a06_treatmentSummary.Rmd
Introduction
The DrugUtilisation package incorporates a function to summarise the
dose table over multiple cohorts: summariseTreatment()
. In
this vignette, we will explore the functionalities and usages of this
function.
Create mock table
library(DrugUtilisation)
library(CDMConnector)
library(dplyr)
library(PatientProfiles)
cdm <- mockDrugUtilisation(numberIndividual = 200)
new_cohort_set <- settings(cdm$cohort1) %>%
dplyr::arrange(cohort_definition_id) %>%
dplyr::mutate(cohort_name = c("asthma","bronchitis","pneumonia"))
cdm$cohort1 <- cdm$cohort1 |>
newCohortTable(cohortSetRef = new_cohort_set)
new_cohort_set <- settings(cdm$cohort2) %>%
dplyr::arrange(cohort_definition_id) %>%
dplyr::mutate(cohort_name = c("albuterol","fluticasone","montelukast"))
cdm$cohort2 <- cdm$cohort2 |>
newCohortTable(cohortSetRef = new_cohort_set)
For the following examples, we will use the cohort
cohort1
and cohort2
already created in the
mock table.
Notice that cohort1
is a cohort with three subcohorts
representing three different conditions:
settings(cdm$cohort1)
#> # A tibble: 3 × 2
#> cohort_definition_id cohort_name
#> <int> <chr>
#> 1 1 asthma
#> 2 2 bronchitis
#> 3 3 pneumonia
And cohort2
is a cohort with three different
treatments:
settings(cdm$cohort2)
#> # A tibble: 3 × 2
#> cohort_definition_id cohort_name
#> <int> <chr>
#> 1 1 albuterol
#> 2 2 fluticasone
#> 3 3 montelukast
Summarise treatment with summariseTreatmentFromCohort() function
summariseTreatmentFromCohort()
function creates a
standarised tibble summarising the treatment information (already
specified in an existing cohort) over multiple cohorts. There are three
mandatory arguments:
-
cohort
: cohort from the cdm object. -
treatmentCohortName
: name of the treatment cohort’s table. -
window
: list of the windows where to summarise the treatments.
See an example of its usage below, where we use
summariseTreatmentFromCohort()
to summarise treatments
defined in cohort2
in the cohorts defined in
cohort1
.
summariseTreatmentFromCohort(cohort = cdm$cohort1,
treatmentCohortName = c("cohort2"),
window = list(c(0,0), c(1,30)))
#> # A tibble: 48 × 13
#> result_id cdm_name group_name group_level strata_name strata_level
#> <int> <chr> <chr> <chr> <chr> <chr>
#> 1 1 DUS MOCK cohort_name asthma overall overall
#> 2 1 DUS MOCK cohort_name asthma overall overall
#> 3 1 DUS MOCK cohort_name asthma overall overall
#> 4 1 DUS MOCK cohort_name asthma overall overall
#> 5 1 DUS MOCK cohort_name asthma overall overall
#> 6 1 DUS MOCK cohort_name asthma overall overall
#> 7 1 DUS MOCK cohort_name asthma overall overall
#> 8 1 DUS MOCK cohort_name asthma overall overall
#> 9 1 DUS MOCK cohort_name asthma overall overall
#> 10 1 DUS MOCK cohort_name asthma overall overall
#> # ℹ 38 more rows
#> # ℹ 7 more variables: variable_name <chr>, variable_level <chr>,
#> # estimate_name <chr>, estimate_type <chr>, estimate_value <chr>,
#> # additional_name <chr>, additional_level <chr>
strata parameter
We can also stratify our cohort and calculate the estimates within
each strata group by using the strata
parameter.
cdm[["cohort1"]] <- cdm[["cohort1"]] %>%
addSex() %>%
addAge(ageGroup = list("<40" = c(0, 39), ">40" = c(40, 150)))
summariseTreatmentFromCohort(cohort = cdm$cohort1,
treatmentCohortName = c("cohort2"),
window = list(c(0,0)),
treatmentCohortId = 1,
strata = list("sex" = "sex", "age" = "age_group")
)
#> # A tibble: 60 × 13
#> result_id cdm_name group_name group_level strata_name strata_level
#> <int> <chr> <chr> <chr> <chr> <chr>
#> 1 1 DUS MOCK cohort_name asthma age_group <40
#> 2 1 DUS MOCK cohort_name asthma age_group <40
#> 3 1 DUS MOCK cohort_name asthma age_group <40
#> 4 1 DUS MOCK cohort_name asthma age_group <40
#> 5 1 DUS MOCK cohort_name asthma age_group >40
#> 6 1 DUS MOCK cohort_name asthma age_group >40
#> 7 1 DUS MOCK cohort_name asthma age_group >40
#> 8 1 DUS MOCK cohort_name asthma age_group >40
#> 9 1 DUS MOCK cohort_name asthma overall overall
#> 10 1 DUS MOCK cohort_name asthma overall overall
#> # ℹ 50 more rows
#> # ℹ 7 more variables: variable_name <chr>, variable_level <chr>,
#> # estimate_name <chr>, estimate_type <chr>, estimate_value <chr>,
#> # additional_name <chr>, additional_level <chr>
Notice that we have also used the treatmentCohortId
parameter to specify that we only want to explore albuterol
across the cohorts defined in cohort1
.
Summarise treatment with
summariseTreatmentFromConceptSet()
function
summariseTreatmentFromCohort()
can be used when we have
already created our treatment cohort. However, if that is not the case,
we can use summariseTreatmentFromConceptSet()
to avoid
creating a new cohort before using this function. The following
arguments have to be provided:
-
cohort
: cohort from the cdm object. -
treatmentConceptSet
: list with the treatments’ concepts sets. -
window
: list of the windows where to summarise the treatments.
Unfortunately, is not implemented yet. Stay in the loop for the next release!😉