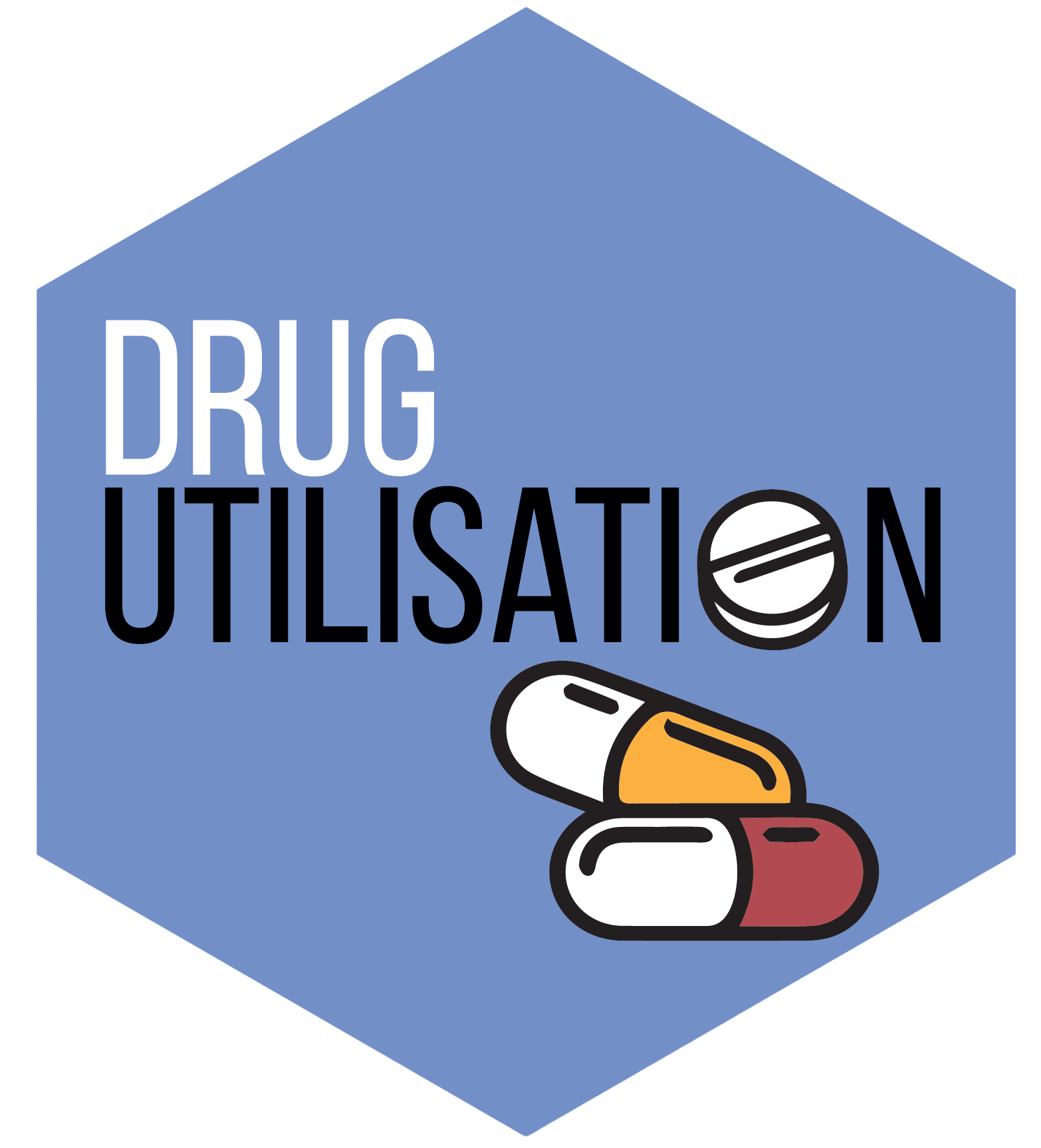
Generate a plot visualisation (ggplot2) from the output of summariseIndication
Source:R/plots.R
plotIndication.Rd
Generate a plot visualisation (ggplot2) from the output of summariseIndication
Usage
plotIndication(
result,
facet = cdm_name + cohort_name ~ window_name,
colour = "variable_level"
)
Examples
# \donttest{
library(DrugUtilisation)
library(CDMConnector)
cdm <- mockDrugUtilisation()
indications <- list(headache = 378253, asthma = 317009)
cdm <- generateConceptCohortSet(cdm = cdm,
conceptSet = indications,
name = "indication_cohorts")
cdm <- generateIngredientCohortSet(cdm = cdm,
name = "drug_cohort",
ingredient = "acetaminophen")
#> ℹ Subsetting drug_exposure table
#> ℹ Checking whether any record needs to be dropped.
#> ℹ Collapsing overlaping records.
#> ℹ Collapsing records with gapEra = 1 days.
result <- cdm$drug_cohort |>
summariseIndication(
indicationCohortName = "indication_cohorts",
unknownIndicationTable = "condition_occurrence",
indicationWindow = list(c(-Inf, 0), c(-365, 0))
)
#> ℹ Intersect with indications table (indication_cohorts)
#> ℹ Summarising indications.
plotIndication(result)
# }